8.1. Hello World (Python)#
While eCAL is written in C++, it also offers a great python integration. Again, we are going to explore that integration by implementing a Hello World Application, that sends the string “Hello World” to an eCAL topic.
Important
The eCAL Python integration is called python3-ecal5
.
With the 5
at the end.
The other name has already been taken by another package.
8.1.1. Dependencies#
First, you have to install some more development dependencies:
Windows:
Python 3 64-bit (https://www.python.org/downloads/)
The eCAL python integration. Choose the appropiate version from the All releases and download the
.whl
file. Open a command prompt in your donwload directory and install the python whl with:pip install ecal5-****-win_amd64.whl
Ubuntu:
Python 3:
sudo apt install python3 python3-pip
The eCAL Python integration.
If you have installed eCAL from a PPA:
sudo apt install python3-ecal5
If you are not using a PPA, choose the appropiate version from the All releases and download the
.whl
file. Open a terminal in your donwload directory and install the python whl with:sudo pip3 install ecal5-*-linux_x86_64.whl
8.1.2. Hello World Publisher#
Somewhere on your hard drive create an empty directory and create a file hello_world.py
with the following content:
hello_world.py
:1import sys 2import time 3 4import ecal.core.core as ecal_core 5from ecal.core.publisher import StringPublisher 6 7if __name__ == "__main__": 8 # initialize eCAL API. The name of our Process will be "Python Hello World Publisher" 9 ecal_core.initialize(sys.argv, "Python Hello World Publisher") 10 11 # Create a String Publisher that publishes on the topic "hello_world_python_topic" 12 pub = StringPublisher("hello_world_python_topic") 13 14 # Create a counter, so something changes in our message 15 counter = 0 16 17 # Infinite loop (using ecal_core.ok() will enable us to gracefully shutdown 18 # the process from another application) 19 while ecal_core.ok(): 20 # Create a message with a counter an publish it to the topic 21 current_message = "Hello World {:6d}".format(counter) 22 print("Sending: {}".format(current_message)) 23 pub.send(current_message) 24 25 # Sleep 500 ms 26 time.sleep(0.5) 27 28 counter = counter + 1 29 30 # finalize eCAL API 31 ecal_core.finalize()
Note
What is happening here?
Line 4-5 imports the eCAL Core and the eCAL String publisher (In this tutorial we only want to send raw strings). eCAL supports multiple message formats.
Line 9 Initialized the eCAL API. The name of our eCAL Process will be “Python Hello World Publisher”. This name will be visible in the eCAL Monitor, once the Process is running.
Line 13 creates an eCAL Publisher. An eCAL Process can create multiple publishers (and multiple subscribers). The topic we are publishing on will be “hello_world_python_topic”.
line 19 Creates an infinite publish-loop. eCAL supports a stop-signal; when an eCAL Process is stopped,
ecal_core.ok()
will return false.Line 23 will publish our message and send it to other eCAL Processes that have subscribed on the topic.
Line 31 de-initializes eCAL. You should always do that before your application exits.
Now execute your python hello world script and take a look at the eCAL Monitor! You will see the “Python Hello World Publisher” process and the “hello_world_python_topic”.
Windows:
python hello_world_snd.py
Ubuntu:
python3 hello_world_snd.py
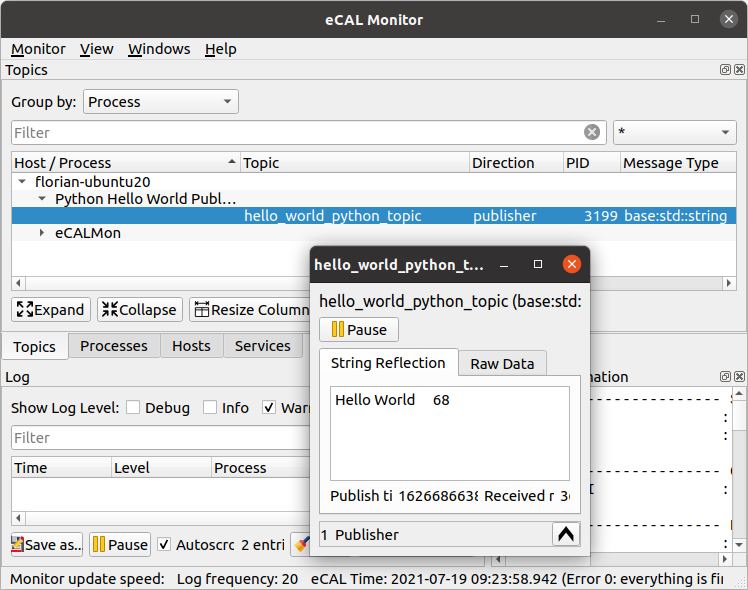
8.1.3. Hello World Subscriber#
For the subscriber, create another file hello_world_rec.py
with the following content:
hello_world_rec.py
:1import sys 2import time 3 4import ecal.core.core as ecal_core 5from ecal.core.subscriber import StringSubscriber 6 7# Callback for receiving messages 8def callback(topic_name, msg, time): 9 print("Received: {}".format(msg)) 10 11if __name__ == "__main__": 12 # Initialize eCAL 13 ecal_core.initialize(sys.argv, "Python Hello World Publisher") 14 15 # Create a subscriber that listenes on the "hello_world_python_topic" 16 sub = StringSubscriber("hello_world_python_topic") 17 18 # Set the Callback 19 sub.set_callback(callback) 20 21 # Just don't exit 22 while ecal_core.ok(): 23 time.sleep(0.5) 24 25 # finalize eCAL API 26 ecal_core.finalize()
Note
What is happening here?
Line 5 Imports the eCAL StringSubscriber class
Line 8-9 Is the receive callback. This method will be called whenever a new message arrives.
Line 16 creates an eCAL subscriber that listens to the “hello_world_python_topic”.
Line 19 adds the receive callback from above to the subscriber, so it can be called.
Important
eCAL Receive callbacks run in the subscriber’s receive thread. While the callback is running, the subscriber cannot receive new data. So, if your callback needs really long to compute, you may have to decouple your computations to not loose messages.
When you now execute hello_world_snd.py
and hello_world_rec.py
, the receiver application will receive the messages sent by the sender.
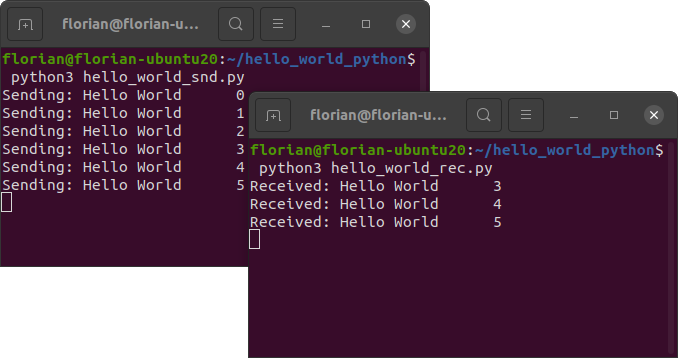
Awesome, you have written your first eCAL publisher and subscriber with python! In general however you will want to have a better way to structure your data than a raw string. So let’s head on to the next topic where we will check out protobuf!
8.1.4. Files#
├─hello_world_snd.py
└─hello_world_rec.py